The new Apple IPad (IPad 3) came out on the 16th, and probably the first thing I noticed about it is how great the screen looks. It’s armed with same “retina” display that the IPhone 4 came out with a few years ago. It’s great! Except for one thing- a lot of images on the web look like crap now!
(This first image is the standard resolution, the second is optimized for IPhone 4 and IPad 3 screens)
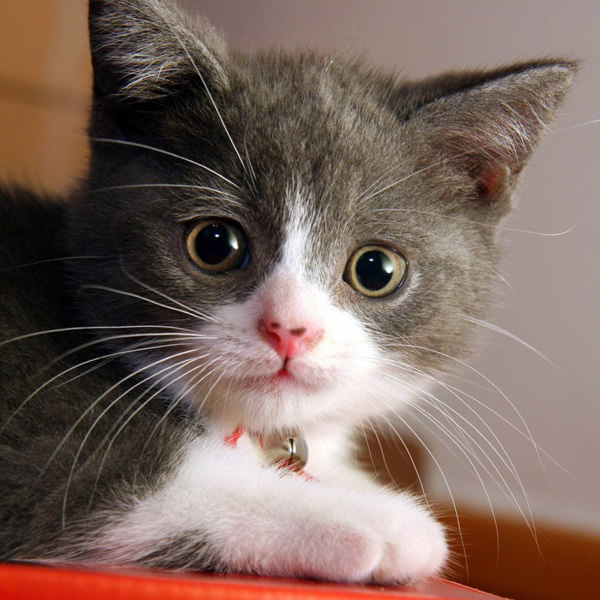
Now- this isn’t new. The IPhone 4 has the same “quirk”, but I think it’s less noticeable given the the size of it’s screen. It really stands out on the IPad 3’s 9.7 inch screen.
The Problem with Pixels
With the advent of high pixel density displays, the pixel itself is now a relative unit.
According to the CSS 2.1 Spec:
Pixel units are relative to the resolution of the viewing device, i.e., most often a computer display. If the pixel density of the output device is very different from that of a typical computer display, the user agent should rescale pixel values.
So, a CSS “pixel” indicates one point on the “virtual” pixel grid to which your CSS design aligns. This either directly matches the actual device, or it is “somehow” scaled to suite.
Talking about the new IPad 3 specifically, the new retina display has a huge 2048 x 1536 pixel resolution- double what most sites are designed for. On a desktop machine, if you doubled your screen resolution, websites would just show up half as big. But on the IPad 3, it stretches the site so it “fills up” the screen. The problem with this is stretching raster images (gif, png, jpeg’s) can make them look really distorted and full of artifacts.
So- how do you fix this?
The easiest way to fix this is to make a second copy of all your images at double the resolution, and then use these versions when visitors are on an IPad or IPhone (or any device that has a higher pixel density).
Fixing it in CSS
The min-device-pixel-ratio media query can be used to target style for high pixel density displays. For the moment vendor prefixes are required, until there is a standard format. For example, Mozilla and Webkit prefixes work the same way, but Opera requires the pixel ratio as a fraction.
-moz-min-device-pixel-ratio: 2
-o-min-device-pixel-ratio: 2/1
-webkit-min-device-pixel-ratio: 2
min-device-pixel-ratio: 2
Right now, we only care about IPhone/IPad, so we’ll use the -webkit-min-device-pixel-ratio tag.
So let’s say you had a single class, loading the 300 x 300 px image:
.logo {
background-image: url(cat_300.jpg);
width: 300px;
height: 300px;
}
You would then create a second copy of the image at 600 x 600 px resolution, and add this in your CSS:
@media only screen and (-webkit-min-device-pixel-ratio: 2) {
.logo {
background-image: url(cat_600.jpg);
background-size: 300px 300px;
}
}
This loads the 600 x 600 px image, but forces the background-size to 300 x 300 px when the device is a webkit device, and the pixel ratio is 2.
Forcing the 600 x 600 px image into a 300 x 300 px box, forces the image to a pixel density of 2.
Fixing Inline Images
So that’s CSS- what about plain old <img> tags?
You can use the window.devicePixelRatio property in JavaScript to determine if the pixel density of the screen is > 1 and if so, cycle through all the images on the page and change their image src to the higher resolution image.
An easy way to do this is to add a class to all the images you want to replace. In this case, I’ve added the “hd” class to the image tags.
<img src="cat_300.jpg" width="300" height="300" class="hd" />
Then add some simple JavaScript to update the tags. In my example, I’ve used jQuery just to make things easier, and simply did a text replace in the src image name, changing the “300” to a 600″. The width/height of the <img> tag needs to stay at 300 x 300 px, forcing the pixel density of 2.
$(document).ready(function()
{
if ( (window.devicePixelRatio) && (window.devicePixelRatio >= 2) )
{
var images = $('img.hd');
for(var i=0; i<images.length; i++)
{
images.eq(i).attr('src', images.eq(i).attr('src').replace('300', '600'))
}
}
});
Now, there are all sorts of ways to do this- this is just one example.
The other way to do this is to just always load the higher resolution images. The only downside, is those higher resolution images are likely almost twice the size of their originals- so loading them only when required will save bandwidth.
What about SVG?
SVG (Scalable Vector Graphics) is also another great way to handle this. SVG files are actually XML files that have instructions on how to “draw” the image on a canvas, rather than using a static raster image. SVG files can scale to different sizes/pixel densities, without distorting.
The only downsides with SVG, is that sometimes the file size for complex images are actually a lot bigger than their raster counterparts, and browser support is still incomplete- so if you care about your site working in Internet Explorer, then you still need to have some raster images and do conditional loading.
Parting Thoughts
Remember- you don’t *actually* need to do any of this; a lot of images will still look “fine” being stretched.
But if you want your site to look it’s best, it’s worth spending the time to optimize it for higher pixel density devices- there’s going to be no shortage of them in the coming years!